Developer API¶
The Kasm Workspaces Developer API can be leveraged to extend the systems functionality and/or integrate with an exiting platform. Integrators interface with the API to create and manage sessions, users and groups.
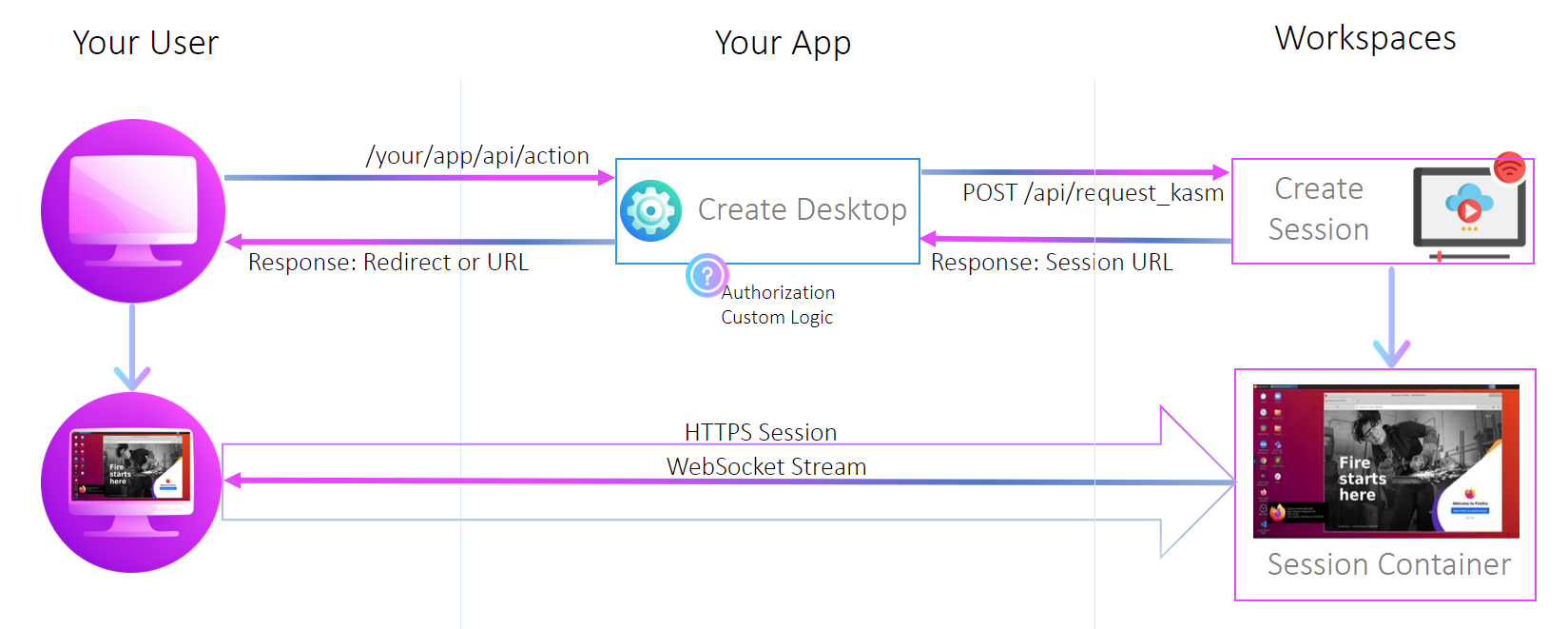
Integration Workflow¶
Code-less Integration Options¶
Integrators are encouraged to review Session Casting which provides an easy way to expose access to Kasm sessions via special URLs.
API Keys¶
API Keys can be generated via the Developers tab. An API_KEY and API_KEY_SECRET will be automatically generated.
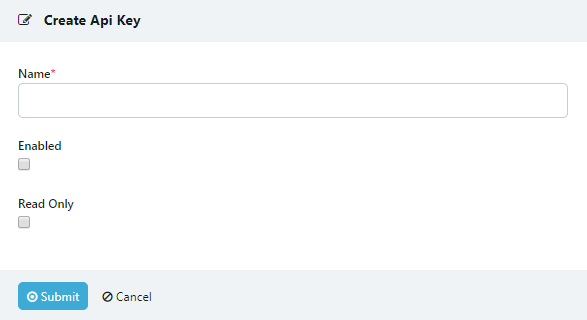
Creating an API Key¶
Authentication¶
The API key and secret must be sent with all of the following requests as json data.
{ "api_key": "bMjMwTT0JKUQ" "api_key_secret": "KUNAvRw4KLHGmldBxhRUD5sAhWkvJVzS" }
Base URL¶
https://<kasm_server>/api/public/
Errors¶
In the event of an error processing an api request, Kasm will respond with an error_message in the body of the json response
Example Response
{"error_message": "<error details>"}
Users¶
Create User¶
Create a new user.
-
POST
/api/public/create_user
¶ Example request:
{ "api_key":"{{api_key}}", "api_key_secret": "{{api_key_secret}}", "target_user": { "username" : "test_user1@example.com", "first_name" : "Bob", "last_name" : "Williams", "locked": false, "disabled": false, "organization": "example", "phone": "123-456-7890", "password": "3UPKGg7g!a9g2@39v6" } }
Example response:
{ "user": { "first_name": "Bob", "username": "test_user1@example.com", "realm": "local", "last_name": "Williams", "phone": "123-456-7890", "last_session": null, "notes": null, "user_id": "50faa5439c574b518cd868dac9256e4b", "groups": [ { "name": "All Users", "group_id": "68d557ac4cac42cca9f31c7c853de0f3" } ], "disabled": false, "organization": "example", "locked": false } }
Get User¶
Retrieve the properties of an existing user. target_user.user_id
or target_user.username
can be passed
-
POST
/api/public/get_user
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "target_user": { "user_id": "4bbb6998064a4d1ea4685f3cdd05feb4", "username": "user@kasm.local" } }
Example response:
{ "user": { "user_id": "4bbb6998064a4d1ea4685f3cdd05feb4", "username": "user@kasm.local", "locked": false, "last_session": "2020-11-09 14:41:33.578622", "groups": [ { "name": "All Users", "group_id": "68d557ac4cac42cca9f31c7c853de0f3" } ], "first_name": null, "last_name": null, "phone": null, "organization": null, "notes": null, "kasms": [ { "kasm_id": "28cfc2a510bb424cad18e571f11ee26c", "start_date": "2020-11-09 14:46:42.321900", "keepalive_date": "2020-11-09 14:46:43.140623", "expiration_date": "2020-11-09 15:46:43.140623", "server": { "server_id": "36e2aeff0e02445db26a521c9c85f1c1", "hostname": "proxy", "port": 443 } } ], "realm": "local", "two_factor": false, "program_id": null, "hash": "9fdfdaad0098cdd8a466b2badbaf962e40214affb07596a6e9d776a409793780" } }
Get Users¶
Retrieve the list of users registered in the system.
-
POST
/api/public/get_users
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", }
Example response:
{ "users": [ { "kasms": [], "company": {}, "username": "user@kasm.local", "locked": false, "realm": "local", "phone": null, "first_name": null, "notes": null, "user_id": "57e8fc1a-fa86-4ff4-9474-60d9831f42d5", "last_session": "2020-11-12 14:29:25.808258", "groups": [ { "name": "All Users", "group_id": "68d557ac4cac42cca9f31c7c853de0f3" } ], "disabled": true, "organization": null, "last_name": null }, { "kasms": [], "company": {}, "username": "admin@kasm.local", "locked": false, "realm": "local", "phone": null, "first_name": null, "notes": null, "user_id": "4acb13bf-1215-4972-9f0d-8c537d17f2da", "last_session": "2020-11-12 14:35:19.700863", "groups": [ { "name": "All Users", "group_id": "68d557ac4cac42cca9f31c7c853de0f3" }, { "name": "Administrators", "group_id": "31aa063c670648589533d3c42092d02a" } ], "disabled": false, "organization": null, "last_name": null } ] }
Update User¶
Update the properties of an existing user.
-
POST
/api/public/update_user
¶ Example request:
{ "api_key":"{{api_key}}", "api_key_secret": "{{api_key_secret}}", "target_user": { "user_id": "4acb13bf-1215-4972-9f0d-8c537d17f2da", "username" : "test_user1@example.com", "first_name" : "Bob", "last_name" : "Williams", "locked": false, "disabled": false, "organization": "example", "phone": "123-456-7890", "password": "3UPKGg7g!a9g2@39v6" } }
Example response:
{ "user": { "first_name": "Bob", "username": "test_user1@example.com", "realm": "local", "last_name": "Williams", "phone": "123-456-7890", "last_session": null, "notes": null, "user_id": "50faa5439c574b518cd868dac9256e4b", "groups": [ { "name": "All Users", "group_id": "68d557ac4cac42cca9f31c7c853de0f3" } ], "disabled": false, "organization": "example", "locked": false } }
Delete User¶
Delete an existing user. If the user has any existing Kasm sessions, deletion will fail. Set the force option to true to delete the user’s sessions and delete the user.
-
POST
/api/public/delete_user
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "target_user": { "user_id": "4bbb6998064a4d1ea4685f3cdd05feb4" }, "force": false }
Example response:
{}
Logout User¶
Logout all sessions for an existing user.
-
POST
/api/public/logout_user
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "target_user": { "user_id": "4bbb6998064a4d1ea4685f3cdd05feb4" } }
Example response:
{}
Get User Attributes¶
Get the attribute (preferences) settings for an existing user.
-
POST
/api/public/get_attributes
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "target_user": { "user_id": "67d7c4e6-f891-4900-897f-ce5ed62fecc0", } }
Example response:
{ "user_attributes": { "ssh_public_key": "ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQCjy8izTD7DlykY0J7iiBn4ysIRqLBwM94ZnjfYH1XAo96ay3grQjSDl5f4u0hrVz6bX62kgPlm9QvTqceNZ+rC/anKp/9nQIrDM6y2W3jNUM6Eo+Ryh7xIOII2lnCbtE/M4urX4lZx3oB2JyIMSIN3yvKUBCSht5FsFabguc8i+nwLvXjmnZx+fcR2/BNcIM9UjCfYBjLFd1XFER7aIXjRy7y2MJQHCFrzhDThNllJ6C1oMZiBsBJ5lXpBmlim80A9IvcW7YBgsAQoqLgrCvRc7IdENTzPAyyhODE/ib5SkwK/peUuCRM+SZnPpUGlv9emLXVjrg5P+TPO/N/v7lyj", "show_tips": false, "user_id": "57e8fc1afa864ff4947460d9831f42d5", "toggle_control_panel": false, "chat_sfx": true, "user_attributes_id": "ef7b72db25b14ab1ac98ac19676ac93f", "default_image": null, "auto_login_kasm": null } }
Update User Attributes¶
Update a users attributes.
-
POST
/api/public/update_user_attributes
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "target_user_attributes": { "user_id": "67d7c4e6-f891-4900-897f-ce5ed62fecc0", "auto_login_kasm": false, "default_image": "b2609fbf72954b20a56c1fe502aa2c41", "show_tips": false, "toggle_control_panel": false } }
Example response:
{}
Kasms¶
Request Kasm¶
Requesting a Kasm will create and start the container and assign a user to that Kasm.
Note
If you intend to integrate Kasm into your app and will utilize iframes to load user sessions, be sure to set the Same Site Cookie Policy Global Setting to None if the Kasm Workspaces server is hosted under a different domain name. Reference: https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie/SameSite
-
POST
/api/public/request_kasm
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "user_id": "8affcdbc16fc4910acb8a6dc268cd7ed", "image_id": "6a2d63fa8959412ca5fddd3890fb7223", "enable_sharing": true, "environment": { "ENV_VAR": "value" } }
Example response:
{ "kasm_id": "6d62ec66-3062-4f1d-b861-aeb3f5ca2390", "session_token": "b03bf84a-53fc-4934-85e4-c434461f1178", "username": "1d47uudwcynkd2su", "status": "starting", "share_id": "fe9770a4", "user_id": "8affcdbc16fc4910acb8a6dc268cd7ed", "kasm_url": "/#/connect/kasm/6d62ec66-3062-4f1d-b861-aeb3f5ca2390/8affcdbc16fc4910acb8a6dc268cd7ed/b03bf84a-53fc-4934-85e4-c434461f1178" }
Arguments
- user_id* string:
An user Id can be specified to create the Kasm under that user. If no User ID is sent an anonymous user will be created and used for the Kasm.
- image_id* string:
An image id can be sent for the desired image. Otherwise the default image sent on the group or user level will be used.
- enable_sharing* boolean:
When the enable sharing flag is set to true the kasm will be created with sharing mode automatically enabled.
- kasm_url* string:
If specified, the browser inside the Kasm session will navigate to this page. This is only applicable if the Image used is Kasm Chrome, Kasm Firefox, or Kasm Tor Browser.
- environment* dict:
Optional environmental variables to inject into the created container.
* Optional
Response Format
- kasm_id string:
Returns the ID for the newly created Kasm.
- session_token string:
Returns the token created for the user used in authorization of the Kasm.
- username string:
Returns the username of the specified or newly created user.
- status string:
Returns the operational status of the Kasm. Will be in Starting, Running or Stopped state.
- share_id string:
Returns the Share id
- user_id string:
Returns the user id of the specified or newly created user
- kasm_url string:
Returns the url path to the specified kasm. This path must be appended to the current Kasm Workspaces server address. It is in the form of /#/connect/kasm/<kasm_id>/<user_id>/<session_token>
Direct the user’s browser to this url to access the Kasm session. This link should not be re-used for different users.
Get Kasm Status¶
After creating a kasm the status can be checked with get_kasm_status. This call also updates the session token for the user creating a new connection link and invalidating the old one.
-
POST
/api/public/get_kasm_status
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "user_id": "c7357f11eb4d47ad8021b5847d8415d8", "kasm_id": "d79ccb22-f6e7-4473-b8a2-a77da82278da" }
Example response:
{ "current_time": "2020-11-12 13:30:24.833834", "kasm_url": "/#/connect/kasm/116f18170fdc4a1a87146164a880fb93/583035bb-5819-4e51-83b9-21223badc569/2d110a49-4c6a-48b9-8cfb-398d6f543d5b", "kasm": { "expiration_date": "2020-11-12 14:28:40.446756", "container_ip": "192.168.32.9", "start_date": "2020-11-12 13:28:40.446737", "point_of_presence": null, "token": "43ab8a765e1e42fe9ee637783731f577", "image_id": "e12266d0f5f44bf5afb80b6c41fabce2", "view_only_token": "6f756f76e8704772bd8e32eb4d7bb835", "cores": 1.0, "hostname": "kasm.server", "kasm_id": "116f18170fdc4a1a87146164a880fb93", "port_map": { "audio": { "port": 443, "path": "desktop/116f1817-0fdc-4a1a-8714-6164a880fb93/audio" }, "vnc": { "port": 443, "path": "desktop/116f1817-0fdc-4a1a-8714-6164a880fb93/vnc" }, "audio_input": { "port": 443, "path": "desktop/116f1817-0fdc-4a1a-8714-6164a880fb93/audio_input" }, "uploads": { "port": 443, "path": "desktop/116f1817-0fdc-4a1a-8714-6164a880fb93/uploads" } }, "image": { "image_id": "e12266d0f5f44bf5afb80b6c41fabce2", "name": "kasmweb/chrome:1.8.0", "image_src": "img/thumbnails/chrome.png", "friendly_name": "Kasm Chrome" }, "is_persistent_profile": false, "memory": 1768000000, "operational_status": "running", "client_settings": { "allow_kasm_audio": false, "idle_disconnect": 20, "lock_sharing_video_mode": true, "allow_persistent_profile": false, "allow_kasm_clipboard_down": false, "allow_kasm_microphone": false, "allow_kasm_downloads": false, "kasm_audio_default_on": false, "allow_point_of_presence": false, "allow_kasm_uploads": false, "allow_kasm_clipboard_up": false, "enable_webp": false, "allow_kasm_sharing": true, "allow_kasm_clipboard_seamless": false }, "container_id": "dbc977159f79e55c466fec52a5b4954b7c26ba88e51937d85a5077d7c79e92e5", "port": 443, "keepalive_date": "2020-11-12 13:28:40.446754", "user_id": "583035bb58194e5183b921223badc569", "persistent_profile_mode": null, "share_id": "30a09d61", "host": "192.168.32.8", "server_id": "8270f8f0acfd4a34a56cc9a9cb7a67d9" } }
Arguments
- user_id string:
The user ID associated with the requested kasm.
- kasm_id string:
The ID of the desired Kasm.
Response Format
- current_time string:
Returns the time at which the call was recieved in UTC.
- kasm json object:
Returns the Kasm object containing all of the current information.
- kasm_url string:
Returns the link path to the specified kasm. This path must be appended to the current Kasm Workspaces server address. It is in the form of /#/connect/kasm/<kasm_id>/<user_id>/<session_token>
Direct the user’s browser to this url to access the Kasm session. This link should not be re-used for different users.
Join Kasm¶
Join Kasm returns the status of the shared kasm and a join url to connect to the Kasm session as a view-only user.
-
POST
/api/public/join_kasm
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "user_id":"c7357f11eb4d47ad8021b5847d8415d8", "share_id":"89cc8cd3" }
Example response:
{ "current_time": "2020-11-12 13:36:16.065711", "session_token": "c9ade890-7693-44bc-a1d4-797bbddf6771", "user_id": "583035bb58194e5183b921223badc569", "kasm": { "port_map": { "vnc": { "port": 443, "path": "desktop/116f1817-0fdc-4a1a-8714-6164a880fb93/vnc" }, "audio": { "port": 443, "path": "desktop/116f1817-0fdc-4a1a-8714-6164a880fb93/audio" } }, "port": 443, "hostname": "kasm.server", "image": { "image_id": "e12266d0f5f44bf5afb80b6c41fabce2", "name": "kasmweb/chrome:1.8.0", "image_src": "img/thumbnails/chrome.png", "friendly_name": "Kasm Chrome" }, "view_only_token": "6f756f76e8704772bd8e32eb4d7bb835", "user": { "username": "anon_2wipg0symxwmm4i1" }, "share_id": "30a09d61", "host": "192.168.32.8", "client_settings": { "allow_kasm_audio": false, "idle_disconnect": 20, "lock_sharing_video_mode": true, "allow_persistent_profile": false, "allow_kasm_clipboard_down": false, "allow_kasm_microphone": false, "allow_kasm_downloads": false, "kasm_audio_default_on": false, "allow_point_of_presence": false, "allow_kasm_uploads": false, "allow_kasm_clipboard_up": false, "enable_webp": false, "allow_kasm_sharing": true, "allow_kasm_clipboard_seamless": false }, "kasm_id": "116f18170fdc4a1a87146164a880fb93" }, "username": "anon_2wipg0symxwmm4i1", "kasm_url": "/#/connect/join/30a09d61/583035bb58194e5183b921223badc569/c9ade890-7693-44bc-a1d4-797bbddf6771" }
Arguments
- user_id* string:
The user ID used to create the join link. If none supplied an anonymous user will be created.
- share_id string:
The share ID of the desired kasm.
* Optional
Response Format
- current_time string:
Returns the time at which the call was received in UTC.
- kasm json object:
Returns the Kasm object containing all of the current information.
- kasm_url string:
Returns the link path to the specified shared kasm. This path must be appended to the current kasm server address. It is in the form of /#/connect/join/<share_id>/<user_id>/<session_token>
Direct the user’s browser to this url to access the Kasm session. This link should not be re-used for different users.
- session_token string:
Returns the token created for the user used in authorization of the Kasm.
- username string:
Returns the username of the specified or newly created user.
- user_id string:
Returns the user ID of the specified or newly created user.
Get Kasms¶
Retrieve a list of live sessions.
-
POST
/api/public/get_kasms
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}" }
Example response:
{ "current_time": "2020-11-12 13:45:33.253299", "kasms": [ { "expiration_date": "2020-11-12 14:28:40.446756", "container_ip": "192.168.32.9", "server": { "port": 443, "hostname": "proxy", "zone_name": "default", "provider": "hardware" }, "user": { "username": "anon_2wipg0symxwmm4i1" }, "start_date": "2020-11-12 13:28:40.446737", "point_of_presence": null, "token": "43ab8a765e1e42fe9ee637783731f577", "image_id": "e12266d0f5f44bf5afb80b6c41fabce2", "view_only_token": "6f756f76e8704772bd8e32eb4d7bb835", "cores": 1.0, "hostname": "proxy", "kasm_id": "116f18170fdc4a1a87146164a880fb93", "port_map": { "audio": { "port": 443, "path": "desktop/116f1817-0fdc-4a1a-8714-6164a880fb93/audio" }, "vnc": { "port": 443, "path": "desktop/116f1817-0fdc-4a1a-8714-6164a880fb93/vnc" }, "audio_input": { "port": 443, "path": "desktop/116f1817-0fdc-4a1a-8714-6164a880fb93/audio_input" }, "uploads": { "port": 443, "path": "desktop/116f1817-0fdc-4a1a-8714-6164a880fb93/uploads" } }, "image": { "image_id": "e12266d0f5f44bf5afb80b6c41fabce2", "name": "kasmweb/chrome:1.8.0", "image_src": "img/thumbnails/chrome.png", "friendly_name": "Kasm Chrome" }, "is_persistent_profile": false, "memory": 1768000000, "operational_status": "running", "client_settings": { "allow_kasm_audio": false, "idle_disconnect": 20, "lock_sharing_video_mode": true, "allow_persistent_profile": false, "allow_kasm_clipboard_down": false, "allow_kasm_microphone": false, "allow_kasm_downloads": false, "kasm_audio_default_on": false, "allow_point_of_presence": false, "allow_kasm_uploads": false, "allow_kasm_clipboard_up": false, "enable_webp": false, "allow_kasm_sharing": true, "allow_kasm_clipboard_seamless": false }, "container_id": "dbc977159f79e55c466fec52a5b4954b7c26ba88e51937d85a5077d7c79e92e5", "port": 443, "keepalive_date": "2020-11-12 13:28:40.446754", "user_id": "583035bb58194e5183b921223badc569", "persistent_profile_mode": null, "share_id": "30a09d61", "host": "192.168.32.8", "server_id": "8270f8f0acfd4a34a56cc9a9cb7a67d9" } ] }
Destroy Kasm¶
Destroy kasm can be called to destroy a Kasm.
-
POST
/api/public/destroy_kasm
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}" "kasm_id": "1a85859d-9d75-45e1-a173-e720472a24f8", "user_id": "4d892bf39321494da9a159178972c147", }
Example response:
{}
Arguments
- user_id string:
The user ID used of the kasm owner.
- kasm_id string:
The ID of the kasm to be destroyed.
Response Format
If empty response is sent the Kasm was successfully deleted.
Keepalive¶
Issue a keepalive to reset the expiration time of a Kasm session. The new expiration time will be updated to reflect the keepalive_expiration Group Setting assigned to the Kasm’s associated user.
-
POST
/api/public/keepalive
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}" "kasm_id": "1a85859d-9d75-45e1-a173-e720472a24f8" }
Example response:
{ "usage_reached": false }
Arguments
- kasm_id string:
The ID of the kasm to issue the expiration update.
Response Format
The response returns a single value usage_reached. If true, the user has exceeded the quota defined be the usage_limit group setting and the expiration time was not reset.
Frame Stats¶
Gets timing statistics for the next processed frame for a Kasm session. A user must be logged in and using a session for this API call to work. The stats include detailed timing stats on the processing of a single frame. Stats include frame analysis, jpeg/webp encoding, time in flight to client, and more.
-
POST
/api/public/get_kasm_frame_stats
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "kasm_id": "1a85859d-9d75-45e1-a173-e720472a24f8", "user_id": "4035b5e67750416cb8d6db14573ea38c", "client": "auto" }
Example response:
{ "frame": { "resx": 1512, "resy": 858, "changed": 10, "server_time": 12}, "clients": [ { "client": "172.18.0.8_1635856777.276370::websocket", "client_time": 0, "ping": 27, "processes": [{"process_name": "scanRenderQ", "time": 0}] } ], "analysis": 2, "screenshot": 2, "encoding_total": 2, "videoscaling": 0, "tightjpegencoder": {"time": 0, "count": 5, "area": 23005}, "tightwebpencoder": {"time": 0, "count": 0, "area": 0} }
Arguments
- kasm_id string:
The ID of the kasm to get the stats for
- user_id string:
The ID of the user the session belongs to
- client* string:
Which client to retrieve stats for: none, auto, all, or <user_websocket_id>. auto is the default and this will automatically select the client side stats for only the owner of the Kasm session. Use none for Server side stats only. Use all to get stats for all websocket clients. Use a KasmVNC websocket id, such as 172.18.0.8_1627985086.157123::websocket, to specify a specific client.
* Optional
Response Format
- resx integer:
Resolution of frame on x axis.
- resy integer:
Resolutino of frame on y axis.
- changed integer:
Percentage of frame that had changes from last frame.
- server_time integer:
Wall clock time to process frame in ms. Many processes are multi-threaded, this stat shows what the actual time was to fully process the frame serverside.
- analysis integer:
Time taken, in ms, to perform pre-processing of the frame to determine changes.
- encoding_total integer:
Time taken, in ms, to perform encoding. A frame is broken down into rects and based on processing power the rects are encoded in a mix of jpeg and webp and multiple threads. This time indicates the total wall clock time taken to perform encoding.
- videoscaling integer:
When in video mode this represents the time taken, in ms, to perform scaling of the frame.
- tightjpegencoder integer:
Total time, in ms, taken to processes all jpeg rects. Processing occurs in parrallel and this is the aggregate runtime of all threads and is thus not wall clock time.
- tightwebpencoder integer:
Total time, in ms, taken to process all webp rects. Processing occurs in parrallel and this is the aggregate runtime of all threads and is thus not wall clock time.
- clients array:
Array of client side stats for each connected client. At this time, ping is the only relevant stat. Ping is the amount of round trip time it takes for comms to the client, divide by two to get one way trip time.
Bottleneck Stats¶
Returns CPU and network bottleneck statistics that are relevant to the Kasm rendering process, KasmVNC. These metrics may be used to determine if rendering performance issues are CPU constraints on the server-side KasmVNC process or network bandwidth constraints. Stats are returned for each connected client. Since Kasm supports sharing sessions, there can be multiple clients connected to a single session.
-
POST
/api/public/get_kasm_bottleneck_stats
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "kasm_id": "1a85859d-9d75-45e1-a173-e720472a24f8", "user_id": "4035b5e67750416cb8d6db14573ea38c" }
Example response:
{ "kasm_user": { "172.18.0.8_1635858087.783615::websocket": [ 9.8, 9.8, 9.7, 9.7 ] } }
Arguments
- kasm_id string:
The ID of the kasm to issue the expiration update.
- user_id string:
The ID of the user that owns the session.
Response Format
The response is JSON data containing an array of kasm user sessions. Each session has a dictionary entry with the key being the KasmVNC identifier for that websocket connection. The value is an array of decimal values ranging from 0 to 10. They represent, in order: CPU, CPU average, network, and network average. The lower the number, the moreconstrained KasmVNC is in being able to keep up with the target framerate.
Screenshot¶
Gets a screenshot of the requested session.
-
POST
/api/public/get_kasm_screenshot
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "kasm_id": "1a85859d-9d75-45e1-a173-e720472a24f8", "user_id": "4035b5e67750416cb8d6db14573ea38c", "width": 300, "height": 300 }
curl https://kasm.example.com/api/public/get_kasm_screenshot -X POST -H 'Content-Type: application/json' -k -d '{ "api_key": "Tqfi8qmFOrkp", "api_key_secret": "nEtNfNkXx46nEoYpJpVug2c9xP4dpqR8", "kasm_id": "95018d17759e4fffbb5670c9d6c412d0", "user_id": "4035b5e67750416cb8d6db14573ea38c", "width": 1000 }' -o /tmp/image.jpg
Arguments
- kasm_id string:
The ID of the kasm to issue the expiration update.
- user_id string:
The ID of the user the session belongs to.
- width* integer:
The width of the image. Default is 300.
- height* integer:
The height of the image. Height is automatically overriden because the apsect ratio is kept and the height is adjusted accordingly.
* Optional
Response Format
The response is a JPEG image.
Exec Command¶
Execute an arbitrary command inside of a user’s session.
-
POST
/api/public/exec_command_kasm
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "kasm_id": "1a85859d-9d75-45e1-a173-e720472a24f8", "user_id": "4035b5e67750416cb8d6db14573ea38c", "exec_config": { "cmd": "xterm -c 'echo hello'", "environment": { "SOME_ENV_VAR": "some value" }, "workdir": "/home/kasm-user", "privileged": false, "user": "root" } }
curl https://kasm.example.com/api/public/exec_command_kasm -X POST -H 'Content-Type: application/json' -k -d '{ "api_k "Tqfi8qmFOrkp", "api_key_secret": "nEtNfNkXx46nEoYpJpVug2c9xP4dpqR8", "kasm_id": "5dff73a8-8b6b-4b8b-bff7-48183a7c757b", "user_id": "4035b5e67750416cb8d6db14573ea38c", "exec_config": { "cmd": "xterm", "environment": { "SOME_ENV_VAR": "value" } } }'
Arguments
- kasm_id string:
The ID of the kasm to issue the expiration update.
- user_id string:
The ID of the user the session belongs to.
- exec_config dict:
Dictionary of execution settings and the command to run.
- exec_config.cmd string:
The command to run within the user’s container.
- exec_config.environment* dict:
Environmental variables to be added to the environment for this exec session.
- exec_config.workdir* string:
Path to working directory for this exec session.
- exec_config.privileged* bool:
Run exec session as privileged.
- exec_config.user* string:
The user to run the command as. By default it runs as the sandboxed normal user account. Specifying ‘root’ as the value will run the command as root inside the container and bypass the sandboxing that Kasm puts in place. The user value should be either root or not specified so that it runs as the sandboxed user, any other setting will result in the command failing.
* Optional
Response Format
- kasm dict:
A dictionary containing details about the container that the command was executed on.
- current_time timestamp:
The date and time the command was executed, in UTC.
Session Component Control¶
The developer may choose to hide the display of certain visual components that normally appear during a Kasm session. This is done by appending the following query arguments to any of the kasm_url response urls generated by the apis above.
Example
/#/connect/join/89cc8cd3/c7357f11eb4d47ad8021b5847d8415d8/070fce1f-86c6-4e05-9b10-935632acb8ce?disable_control_panel=1&disable_tips=1&disable_chat=1&disable_fixed_res=1
Query Arguments
- disable_control_panel=1
Hides the Kasm control panel that is normally used for uploads, downloads etc. Users will be unable to access this functionality.
- disable_tips=1
Stops the tips modal from showing when the user connects to a session.
- disable_chat=1
Hides the chat interface for shared sessions.
- disable_fixed_res=1
By default, shared sessions are forced into a fixed resolution and aspect ratio. When specified this will allow the shared session to operate with a dynamic resolution and aspect ratio.
Images¶
Get Images¶
Retrieve a list of available images.
-
POST
/api/public/get_images
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}" }
Example response:
{ "images": [ { "restrict_to_network": false, "memory": 768000000, "zone_name": null, "x_res": 800, "description": "Single-Application : Chrome", "image_id": "b483f0a4fb6546f79064f9b6759758cb", "persistent_profile_path": null, "friendly_name": "Kasm Chrome", "volume_mappings": {}, "restrict_to_zone": false, "docker_token": null, "persistent_profile_config": {}, "cores": 1.0, "docker_registry": "https://index.docker.io/v1/", "available": true, "run_config": { "hostname": "kasm" }, "imageAttributes": [ { "image_id": "b483f0a4fb6546f79064f9b6759758cb", "attr_id": "11cd3b92d73c4caaa84c70e75190ec25", "name": "vnc", "category": "port_map", "value": "6901/tcp" }, { "image_id": "b483f0a4fb6546f79064f9b6759758cb", "attr_id": "ec1531322190445db70d10c31766503a", "name": "audio", "category": "port_map", "value": "4901/tcp" }, { "image_id": "b483f0a4fb6546f79064f9b6759758cb", "attr_id": "3634ba1144bc4f64a932a561983c64f5", "name": "uploads", "category": "port_map", "value": "4902/tcp" } ], "docker_user": null, "restrict_to_server": false, "enabled": true, "name": "kasmweb/chrome:1.8.0", "zone_id": null, "y_res": 600, "server_id": null, "network_name": null, "exec_config": { "first_launch": { "environment": { "LAUNCH_URL": "" }, "cmd": "bash -c 'google-chrome --start-maximized \"$KASM_URL\"'" }, "go": { "cmd": "bash -c 'google-chrome --start-maximized \"$KASM_URL\"'" } }, "hash": null, "image_src": "img/thumbnails/chrome.png" } ] }
Groups¶
Add User to Group¶
Add a user to an existing group.
-
POST
/api/public/add_user_group
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "target_user": { "user_id": "67d7c4e6-f891-4900-897f-ce5ed62fecc0" }, "target_group": { "group_id": "87fd617577984033be5bd269b4d170c6" } }
Example response:
{}
Remove User from Group¶
Remove a user from an existing group.
-
POST
/api/public/remove_user_group
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "target_user": { "user_id": "67d7c4e6-f891-4900-897f-ce5ed62fecc0" }, "target_group": { "group_id": "87fd617577984033be5bd269b4d170c6" } }
Example response:
{}
Login¶
Get Login¶
Generate a link for the user to login to Kasm without the need to enter a username or password. Redirect the user to the provided url.
-
POST
/api/public/get_login
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "target_user": { "user_id": "67d7c4e6-f891-4900-897f-ce5ed62fecc0" } }
Example response:
{ "url": "https://kasm.server/#/connect/login/dash/57e8fc1afa864ff4947460d9831f42d5/930d6a5d-082e-438a-b5cf-98ae7ca5b67c" }
Licensing¶
Activate¶
License the deployment by submitting an activation key. If valid, the returned license key will automatically be applied to the deployment.
-
POST
/api/public/activate
¶ Example request:
{ "api_key": "{{api_key}}", "api_key_secret": "{{api_key_secret}}", "activation_key": "-----BEGIN ACTIVATION KEY-----eyJ0eXAiOiJ...-----END ACTIVATION KEY-----", "seats" : 10, "issued_to": "ACME Corp" }
Example response:
{ "license": { "license_id": "12e887e9e94a4615849f7963e9c85363", "expiration": "2022-11-06 18:07:08", "issued_at": "2021-11-05 18:07:08", "issued_to": "ACME Corp", "limit": 10, "is_verified": true, "license_type": "Per Concurrent Kasm", "features": { "auto_scaling": true, "branding": true, "session_staging": true, "session_casting": true, "log_forwarding": true, "developer_api": true, "inject_ssh_keys": true, "saml": true, "ldap": true, "session_sharing": true, "login_banner": true, "url_categorization": true, "usage_limit": true }, "sku": "Enterprise" } }
Arguments
- activation_key string:
The activation key provide by Kasm Technologies.
- seats* integer:
The desired number of seats to license the deployment for. If omitted the deployment will be licensed using the maximum number of seats as dictated by the entitlement.
- issued_to* string:
A string representing the organization the deployment is licensed for.
* Optional
Response Format
- license json object:
The detailed information about the license generated.